Hardhat
Prerequisitesβ
Before you begin, ensure you've:
- Set up your wallet
- Funded your wallet with Linea ETH on either the testnet or mainnet
- Set up your environment using Hardhat's recommended instructions.
Create a Hardhat projectβ
To create an empty Hardhat project, run the following commands:
mkdir linea-tutorial
cd linea-tutorial
npm init
npm install --save-dev hardhat
npx hardhat
In the menu that appears, select Create an empty hardhat.config.js
and press Enter.
Hardhat recommends using their plugin, @nomicfoundation/hardhat-toolbox
, which can be downloaded by entering Y
during the project creation process.
You should now have a sample project from Hardhat, the deploys, and test a Lock
contract that locks funds for a set amount of time.
The default script in scripts/deploy.js
locks 0.001 ETH in the contract upon deployment. If you don't want to do this, modify const lockedAmount = hre.ethers.parseEther("0.001");
to const lockedAmount = 0;
Deploy the contractβ
The sample project already includes the deployment script. To deploy on Linea, you'll just need to make a few modifications.
You can use Truffle Dashboard to deploy directly with your MetaMask wallet or deploy with the Hardhat CLI by adding Linea to your hardhat.config.js
.
Use Truffle Dashboardβ
Truffle Dashboard allows you to forgo saving your private keys locally, instead connecting to your MetaMask wallet for deployments. To deploy with Truffle Dashboard using the Hardhat plugin:
-
Install Truffle using the recommended installation procedure
-
Run:
npm install --save-dev @truffle/dashboard-hardhat-plugin
-
Import the plugin into
hardhat.config.js
, with:import "@truffle/dashboard-hardhat-plugin";
or:
require("@truffle/dashboard-hardhat-plugin");
-
Run
truffle dashboard
in your terminal, which will open a window on port24012
. -
Navigate to
localhost:24012
in your browser. Please ensure that your Truffle Dashboard is connected to the Linea testnet or mainnet by connecting your MetaMask wallet to Linea. For reference, the Linea testnet network ID is59140
.
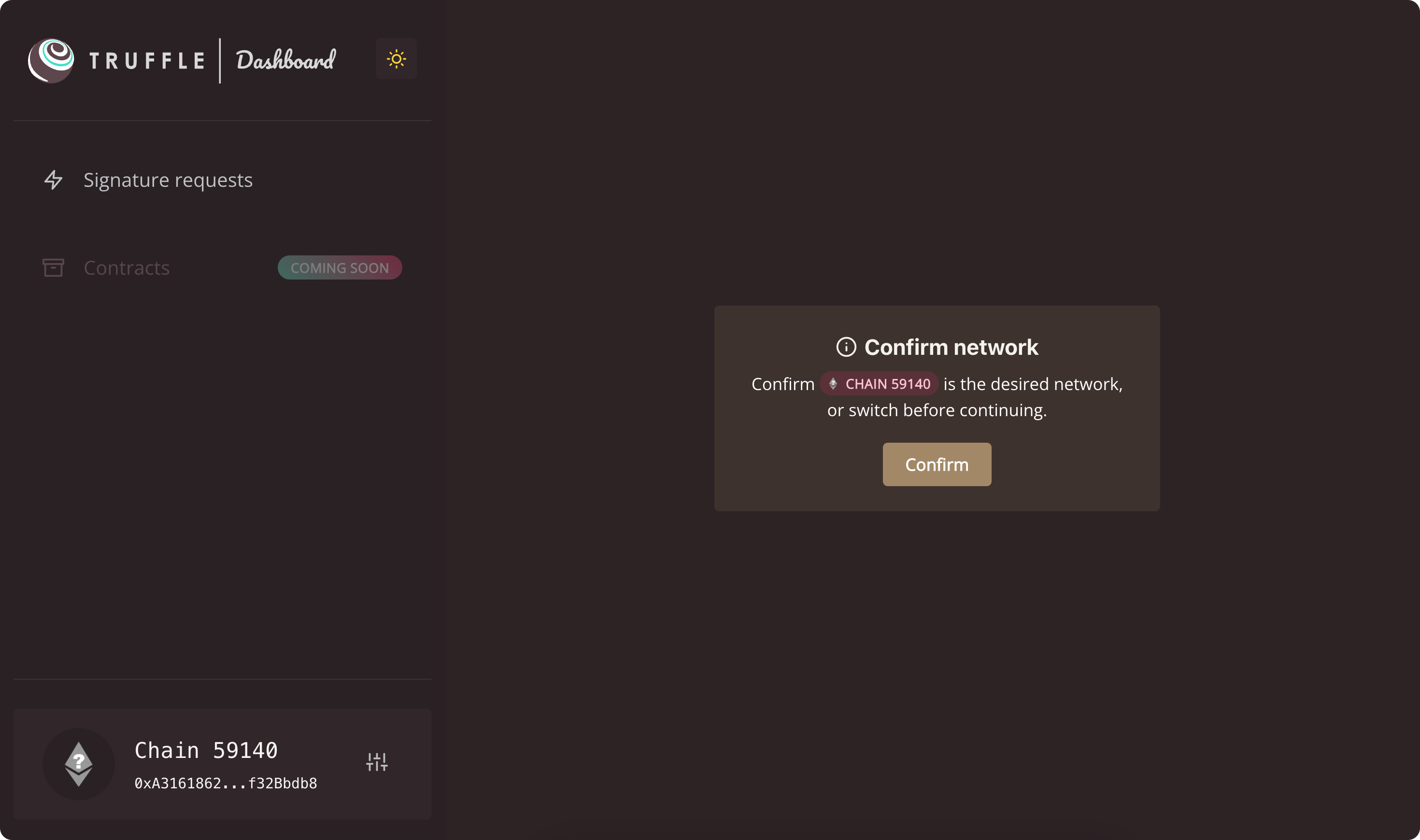
-
In a separate terminal, in your Hardhat project, run
npx hardhat run scripts/deploy.js --network truffleDashboard
-
Navigate back to
localhost:24012
. You should see a prompt asking you to confirm the deployment. Click Confirm.
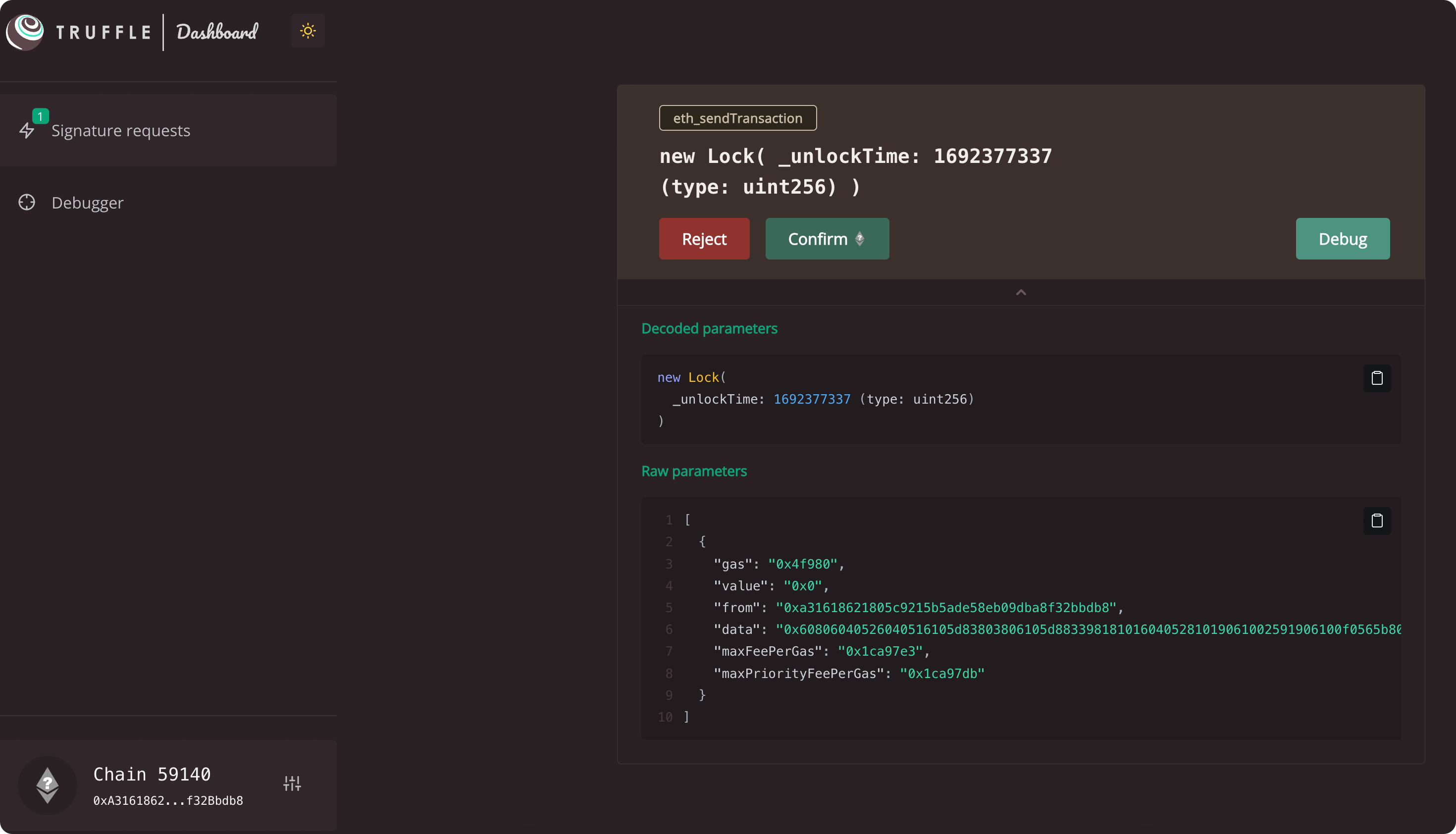
-
You should see something similar to:
Preparing Truffle compilation
Sending Truffle compilation to dashboard
Compilation successfully sent!
Lock with 0.0ETH and unlock timestamp 1692377128 deployed to 0x6230404e79E2082A6454d5b1efF58DacA0C330C7
Use hardhat.config.js
β
To deploy to Linea, we'll need to add the network to our hardhat.config.js
. To do this:
-
Create a
.env
file in the root folder with your wallet's private key.PRIVATE_KEY=YOUR_PRIVATE_KEY
warningPlease do not check your keys into source control. Please add
.env
to your.gitignore
-
Download
dotenv
npm i -D dotenv
-
Add Linea to your
hardhat.config.js
file.
- Infura
- Public Endpoint
To use Infura, you'll need to get an API key. Add it to the .env
file as follows:
PRIVATE_KEY=YOUR_PRIVATE_KEY
INFURA_API_KEY=INFURA_API_KEY
Then, modify the hardhat.config.js like so:
require("@nomicfoundation/hardhat-toolbox");
require("dotenv").config();
const { PRIVATE_KEY, INFURA_API_KEY } = process.env;
module.exports = {
solidity: "0.8.19",
networks: {
linea_testnet: {
url: `https://linea-goerli.infura.io/v3/${INFURA_API_KEY}`,
accounts: [PRIVATE_KEY],
},
linea_mainnet: {
url: `https://linea-mainnet.infura.io/v3/${INFURA_API_KEY}`,
accounts: [PRIVATE_KEY],
},
},
};
The public endpoints are rate limited and not meant for production systems.
require("@nomicfoundation/hardhat-toolbox");
require("dotenv").config();
const { PRIVATE_KEY } = process.env;
module.exports = {
solidity: "0.8.17",
networks: {
linea_testnet: {
url: `https://rpc.goerli.linea.build/`,
accounts: [PRIVATE_KEY],
},
linea_mainnet: {
url: `https://rpc.linea.build/`,
accounts: [PRIVATE_KEY],
},
},
};
- Call
npx hardhat run scripts/deploy.js --network linea_testnet
ornpx hardhat run scripts/deploy.js --network linea_mainnet
from the CLI.
Your output should look something like this:
Lock with 0.0ETH and unlock timestamp 1692381910 deployed to 0xaD6C134BD9EA1E174CC8EA77755BF79C86148d38
Next, you can optionally verify your contract on the network.